插件
Picker
@nativescript/picker
一个 NativeScript 插件,提供一个 UI 元素,用于从以模态弹出窗口打开的列表中选择对象/值。
安装
npm install @nativescript/picker
用法
![]() | ![]() |
---|---|
iOS | Android |
用法
核心
使用 <Page>
视图的 xmlns
属性注册插件命名空间。
<Page
xmlns="http://schemas.nativescript.org/tns.xsd"
xmlns:picker="@nativescript/picker">
<picker:PickerField hint="Click here" items="{{ pickerItems }}"/>
...
或使用项目模板
<picker:PickerField focusOnShow="true" filterKeyName="name" showFilter="{{ enableFilter }}" pickerTitle="Nativescript Picker" rowHeight="60" id="picker" hint="Click here" textField="name" padding="10" pickerOpened="{{ pickerOpened }}" pickerClosed="{{ pickerClosed }}"
items="{{ pickerItems }}" >
<picker:PickerField.itemTemplate>
<GridLayout height="60">
<Label text="{{ name}}" textWrap="true" verticalAlignment="center" />
</GridLayout>
</picker:PickerField.itemTemplate>
</picker:PickerField>
数据过滤器
可以通过设置 showFilter="true"
来过滤数据,默认情况下,插件将查看项目源的 name
键,但可以通过设置 filterKeyName="title"
来控制这一点,假设您的数据项包含一个 title 键。
通过设置
focusOnShow="true"
使搜索栏获得焦点
let dataItems = new ObservableArray<{ title: string; age: number }>()
for (let i = 0; i <= 30; i++) {
dataItems.push({
title: 'Title' + i,
age: 30 + i,
})
}
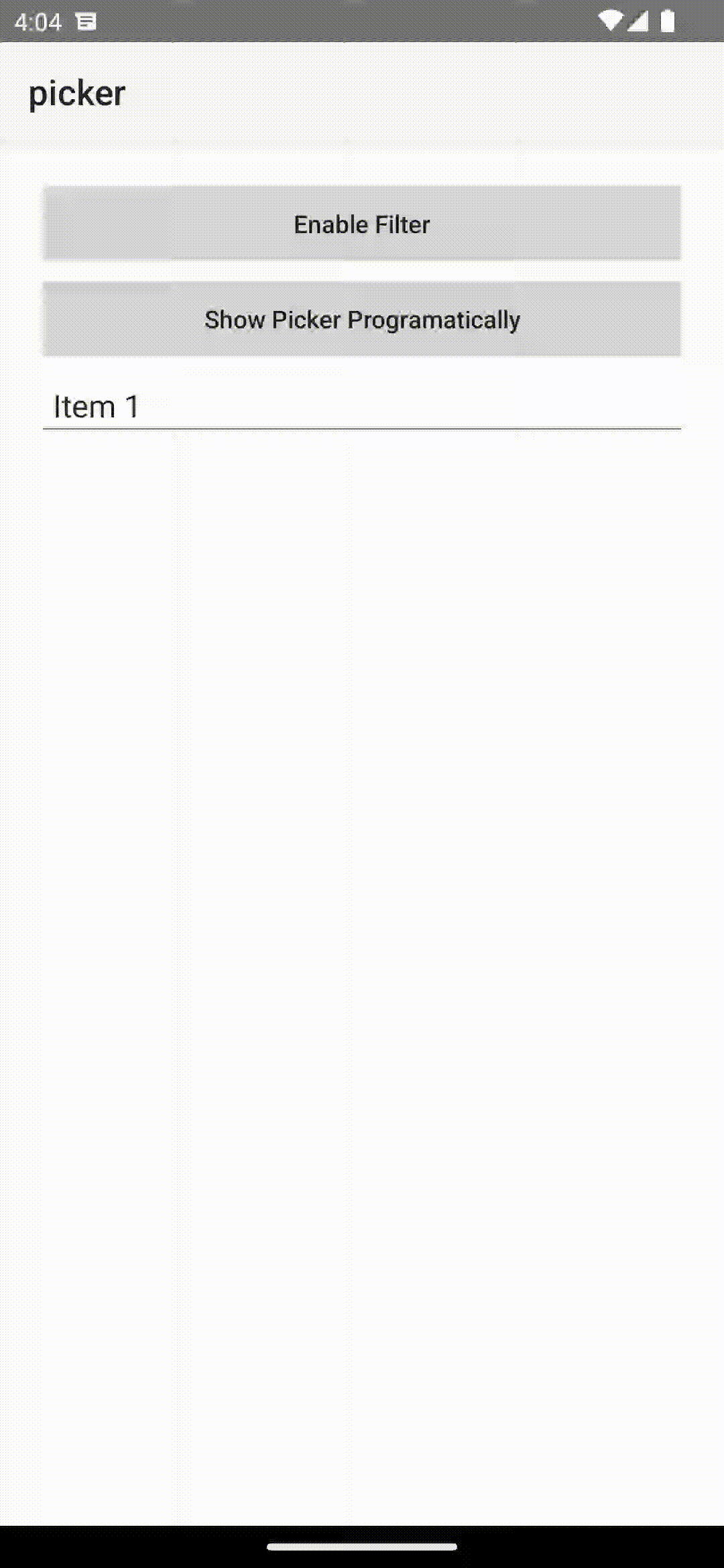
获取选定项目
您需要从 pickerClosed 属性 pickerClosed="onSelectedItem"
注册回调,这将返回 selectedIndex
onSelectedItem(args) {
let index = args.object?.selectedIndex;
console.log('Picker > closed', index);
console.log('Picker > closed', dataItems[index].title);
}
样式
您可以通过这些 css 类来定位 Picker,例如:.pickerRootModal
pickerRootModal
定位Modal
pickerPage
定位Page
pickerGridLayout
定位包含所有视图的GridLayout
包装器pickerListView
定位ListView
pearchBarContainer
定位搜索栏容器StackLayout
pickerSearchBar
定位搜索栏TextField
Angular
在组件的模块中导入插件模块
import { NativeScriptPickerModule } from "@nativescript/picker/angular";
...
@NgModule({
imports: [
NativeScriptPickerModule,
...
],
...
然后在组件的 HTML 中声明字段
<PickerField hint="Click here" [items]="pickerItems"></PickerField>
定义自定义项目模板
您也可以为 Picker 的列表定义自定义项目模板
<PickerField
hint="Click here"
class="picker-field"
textField="name"
[pickerTitle]="'Select item from list'"
[items]="items"
>
<ng-template let-item="item">
<GridLayout columns="auto, *" rows="auto, *">
<label text="Static text:" col="0"></label>
<label [text]="item?.name" col="0" row="1"></label>
<image [src]="item?.imageUrl" col="1" row="0" rowSpan="2"></image>
</GridLayout>
</ng-template>
</PickerField>
使用以下绑定
interface IDataItem {
name: string
id: number
description: string
imageUrl: string
}
this.items = new ObservableArray<IDataItem>()
for (let i = 0; i < 20; i++) {
this.items.push({
name: 'Item ' + i,
id: i,
description: 'Description ' + i,
imageUrl: 'https://picsum.photos/150/70/?random',
})
}
Vue
import PickerField from '@nativescript/picker/vue'
app.use(PickerField)
然后,在组件的模板中声明字段
<PickerField hint="Click here"></PickerField>
样式化 PickerField
PickerField
可以通过其元素选择器以及通过设置类在 CSS 中定位。PickerField
还打开一个模态窗口,其中包含一个 Page 元素,该元素包含一个 ActionBar
和一个 ListView.
此 Page 元素可以使用 PickerPage
选择器定位,如下所示
PickerPage {
}
,并且通过 PickerPage
选择器,您可以使用 PickerPage ActionBar
和 PickerPage ListView
之类的选择器来设置所有 Picker 模态的样式。
PickerPage ActionBar {
}
PickerPage ListView {
}
除此之外,如果您在 PickerField
上设置了一个类,它将被传递到 PickerPage
,并且您可以使用它来设置单个模态的样式。
PickerField API
PickerField
扩展了 TextField
视图,这意味着默认 TextField
提供的任何功能在 PickerField
组件中也可用。唯一的区别是,在设计上它是“只读”模式,或者简单地说,您无法更改其文本。当用户从列表中点击一个值时,会更改 PickerField
的文本。
属性 | 类型 | 描述 |
---|---|---|
itemLoadingEvent | string | 连接到 itemLoading 事件时使用的字符串值。 |
pickerTitle | string | 模态视图的标题。 |
items | items: any[] | ItemsSource | 用于填充模态视图列表的源集合。 |
itemTemplate | string | Template | 模态视图列表的 ListView 项目的 UI 模板。 |
modalAnimated | boolean | 可选参数,指定是否以动画形式显示模态视图。 |
textField | string | 来自 'items' 集合的对象的 '属性',将被 'items' 集合的 'text' 属性使用。 |
valueField | string | 来自 'items' 集合的对象的 '属性',将被用于设置 PickerField 的 selectedValue 属性。 |
selectedValue | any | 从模态视图列表中选定的对象。 |
selectedIndex | number | 来自 items 集合的对象的索引,该对象已从模态视图列表中选中。 |
iOSCloseButtonPosition | 'left' | 'right' | 模态视图 ActionBar 的“关闭”按钮的位置。 |
iOSCloseButtonIcon | number | 模态视图 ActionBar 的“关闭”按钮的图标。 |
androidCloseButtonPosition | 'navigationButton' | 'actionBar' | 'actionBarIfRoom' | 'popup' | 模态视图 ActionBar 的“关闭”按钮的位置。 |
androidCloseButtonIcon | string | 模态视图 ActionBar 的“关闭”按钮的图标。 |
showFilter | 显示搜索栏 | |
filterKeyName | 设置过滤时要使用的对象键(请参阅文档) | |
focusOnShow | 将焦点设置到搜索栏 | |
hintText | 设置搜索栏的提示 |