UI 组件
TextView
用于多行文本输入的 UI 组件。
<TextView>
是一个用于多行文本输入的 UI 组件。设置为只读时,它也可以用于显示多行文本。
对于单行文本输入,请参阅 TextField.
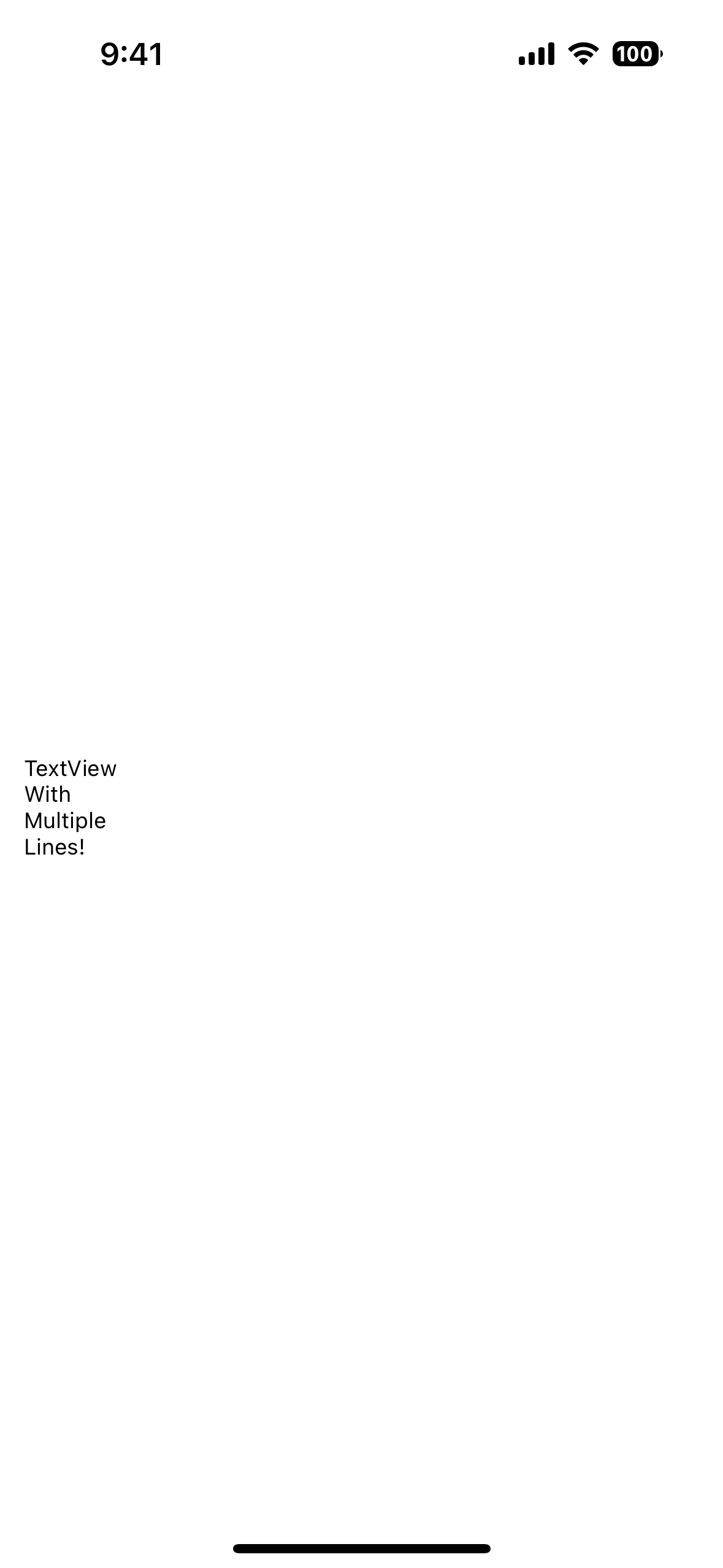
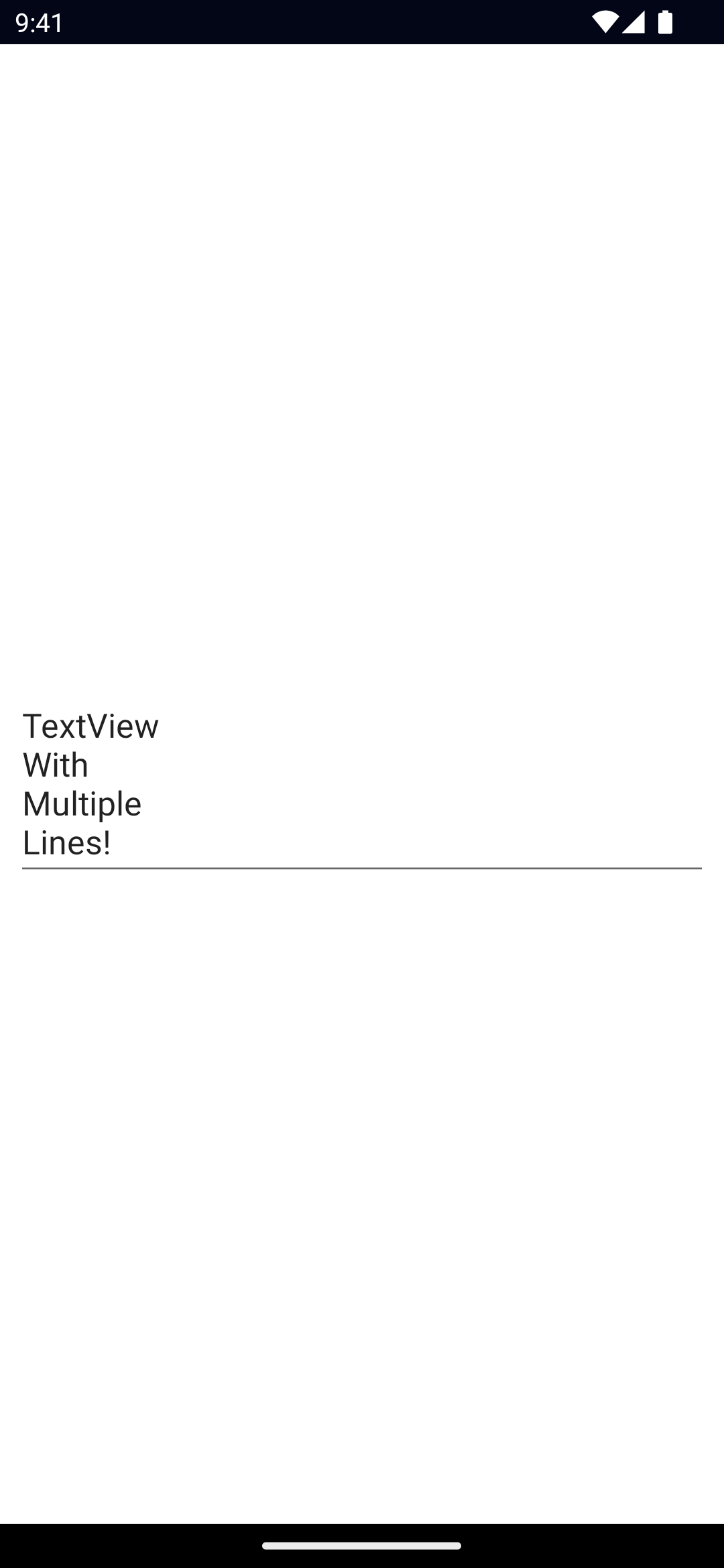
xml
<TextView text="{{ text }}" />
ts
const text = `TextView\nWith\nMultiple\nLines!`
示例
在 TextView 中格式化文本
如果您需要对文本的某些部分进行样式设置,可以使用 FormattedString
和 Span
元素的组合。
xml
<TextView>
<FormattedString>
<Span text="This text has a " />
<Span text="red " style="color: red" />
<Span text="piece of text. " />
<Span text="Also, this bit is italic, " fontStyle="italic" />
<Span text="and this bit is bold." fontWeight="bold" />
</FormattedString>
</TextView>
属性
text
ts
text: string
获取或设置 TextView 的文本。
hint
ts
hint: string
获取或设置 TextView 的占位符文本。
editable
ts
editable: boolean
设置为 false
时,TextView 为只读。
默认值为 true
。
keyboardType
ts
keyboardType: CoreTypes.KeyboardType | number // "datetime" | "email" | "integer" | "number" | "phone" | "url"
获取或设置在编辑此 TextView 时显示的键盘类型。
在 iOS 上,任何有效的 UIKeyboardType
编号都适用,例如
ts
keyboardType = 8 // UIKeyboardType.DecimalPad
请参阅 CoreTypes.KeyboardType,UIKeyboardType.
returnKeyType
ts
returnKeyType: CoreTypes.ReturnKeyType // "done" | "go" | "next" | "search" | "send"
获取或设置返回键的标签。
isEnabled
允许禁用 TextView。禁用的 TextView 不会对用户手势或输入做出反应。
默认值为 true
。
maxLines
ts
maxLines: number
将输入限制为指定的行数。
autocorrect
ts
autocorrect: boolean
启用或禁用自动更正。
...继承
有关未显示的其他继承属性,请参阅 API 参考.
方法
focus()
ts
focus(): boolean
将焦点设置到 TextView 并返回 true
(如果焦点设置成功)。
dismissSoftInput()
ts
dismissSoftInput(): void
隐藏屏幕键盘。
事件
textChange
ts
on('textChange', (args: PropertyChangeData) => {
const textView = args.object as TextView
console.log('TextView text changed:', args.value)
})
当输入文本发生更改时发出。
事件数据类型:PropertyChangeData
returnPress
ts
on('returnPress', (args: EventData) => {
const textView = args.object as TextView
console.log('TextView return key pressed.')
})
当按下返回键时发出。
focus
ts
on('focus', (args: EventData) => {
const textView = args.object as TextView
console.log('TextView has been focused')
})
当 TextView 获得焦点时发出。
blur
ts
on('blur', (args: EventData) => {
const textView = args.object as TextView
console.log('TextView has been blured')
})
当 TextView 失去焦点时发出。
原生组件
- Android:
android.widget.EditText
- iOS:
UITextView
- 上一个
- TextField
- 下一个
- TimePicker